You can add subscribers to any campaign by directly calling EarlyParrot API as follows.
Method 1: Call subscribe API directly from JavaScript
Method: POST
Param:
var ep_subscribeObj = new Object(); ep_subscribeObj.firstName = 'Gaetano'; // ' ' if not available ep_subscribeObj.lastName = 'Caruana'; // ' ' if not available ep_subscribeObj.email = '[email protected]' // Mandatory
ep_subscribeObj.rh = Cookies.get('rh');
URL: https://admin.earlyparrot.com/api/campaigns/[YOUR_CAMPAIGN_ID]/subscribe
Both if the supplied email is a subscriber or not, you will get the following JSON response:
{ ... "user": { "_id": "5a5b28f21b2f10001f6e6c20", "profileImageURL": "https://lh3.googleusercontent.com/-CLCe_GG_Mbo/AAAAAAAAAAI/AAAAAAAAABA/WfAB5P5zNoE/photo.jpg?sz=500", "confirmationToken": "c505a5c561fa4eaa5ca0eaa7cc4110ed5a5b28f21b2f10001f6e6c20", ... "username": "gaetano", "displayName": "Gaetano Caruana", "welcomeEmailSent": false, ... "email": "[email protected]", ... "id": "5a5b28f21b2f10001f6e6c20", ... }, "sharePage": "[YOUR_SHARE_PAGE_URL]?confirm-subscribe=c505a5c561fa4eaa5ca0eaa7cc4110ed5a5b28f21b2f10001f6e6c20" }
The confirmationToken can be persisted as it will never change for a given subscriber.
It is completely safe to call subscribe call multiple times. EarlyParrot will make sure not to duplicate your subscribers but it will simply return the information about the subscriber.
Method 2: Using Google Tag Manager to call add subscriber call
Google Tag Manager will allow you to install, test, deploy and even rollback scripts with ease. Once you have Google Tag Manager installed, you are ready to install and deploy EarlyParrot scripts including binding to a form to capture new sign-ups. Log into your Google Tag Manager and go to Triggers tab.
Create a new trigger new Page View trigger.
Call it EarlyParrot Subscriber Page Trigger. Set the trigger condition to make sure it triggers only on the page that has the sign-up form on it.
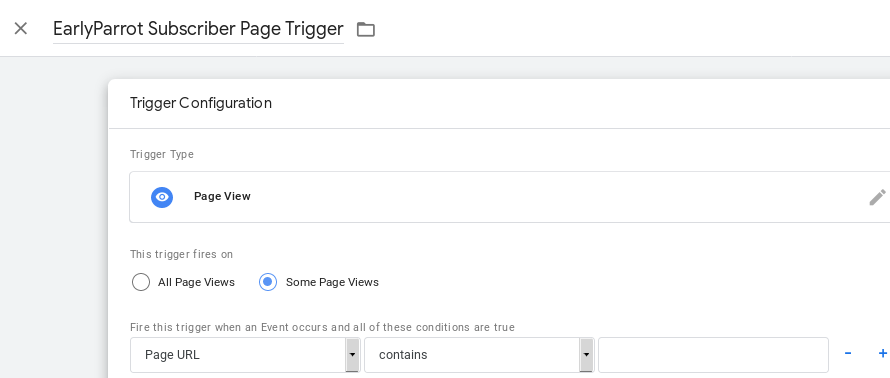
Go to Triggers tab again.
Create a new trigger new Event Trigger.
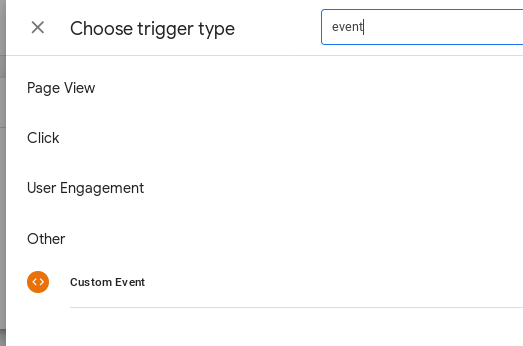
Call it subscribeCallUtilitiesLoaded.
Then go the tags tab.
Create a new Custom HTML tag.
Call it EarlyParrot Subscribe Call Step1 and paste the following script.
<script language="javascript">
(function(w,d,url){
var headElement=d.getElementsByTagName('head')[0];
var scriptElement=d.createElement('script');
scriptElement.addEventListener('load', function() {
window.dataLayer.push({
event: 'subscribeCallUtiltiesLoaded'
});
});
scriptElement.async=1;
scriptElement.src=url
headElement.appendChild(scriptElement);
})(window,document,'https://s3.amazonaws.com/earlyparrot-production-scripts/utilities.js');
</script>
This script will load required utilities.js file. Once this tag has been added go ahead and add another set the trigger to EarlyParrot Subscriber Page Trigger.
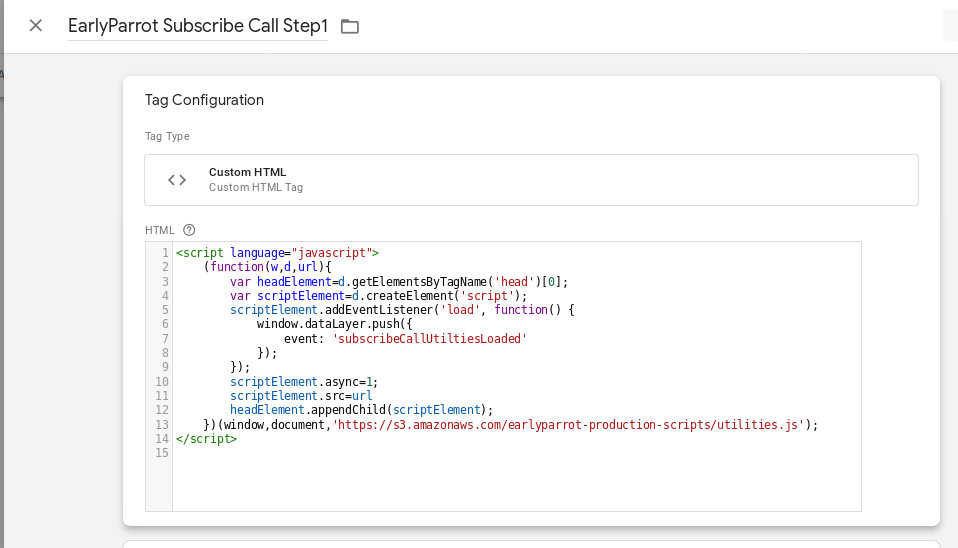
Create another Custom HTML tag and call it EarlyParrot Subscribe Call Step2. Place this snippet of code in the custom HTML tag you just created. Now this snippet of code needs some editing.
- Use JQuery to capture the value of first name, last name and email. Both first name and last name are optional but email is mandatory.
- replace your [YOUR_CAMPAIGN_ID] with your own CampaignId
If you require any assitance to modify the script please do get in touch with us on [email protected]. Our software developers will help you out.
<script language="javascript">
epJQuery('form').submit(function(e) {
var ep_subscribeObj = new Object();
epJQuery("input[name='first_name']").each(function(index, value) {
if (epJQuery(value).val() != null && epJQuery(value).val().length > 0) {
ep_subscribeObj.firstName = epJQuery(value).val();
}
});
epJQuery("input[name='last_name']").each(function(index, value) {
if (epJQuery(value).val() != null && epJQuery(value).val().length > 0) {
ep_subscribeObj.lastName = epJQuery(value).val();
}
});
epJQuery("input[name='email']").each(function(index, value) {
if (epJQuery(value).val() != null && epJQuery(value).val().length > 0) {
ep_subscribeObj.email = epJQuery(value).val();
}
});
ep_subscribeObj.rh = Cookies.get('rh');
ep_subscribeObj.sendWelcomeImmediately = 0; //will not send welcome email
Cookies.set('epSubmitEmail', ep_subscribeObj.email, { domain: 'yourdomain.com' });
epJQuery.post("https://admin.earlyparrot.com/api/campaigns/[YOUR_CAMPAIGN_ID]/subscribe", ep_subscribeObj)
.then(function(data) {
//JSON object returned by subscribe call
var userFirstName = data.user.firstName;
var userLastName = data.user.lastName;
var userEmail = data.user.email;
var sharePageURL = data.sharePage;
var confirmationToken = data.user.confirmationToken;
var domain=document.domain;
var p=domain.split('.');
var i=0;
var current_domain='';
for (var i=0;i<p.length-1;i++) {
current_domain = p.slice(i).join('.');
Cookies.set('confirmToken', confirmationToken, { domain: current_domain });
}
window.dataLayer.push({
'userFirstName': userFirstName,
'userLastName': userLastName,
'userEmail': userEmail,
'sharePageURL': sharePageURL
});
});
});
</script>
Set the trigger to subscribeCallUtilitiesLoaded event.
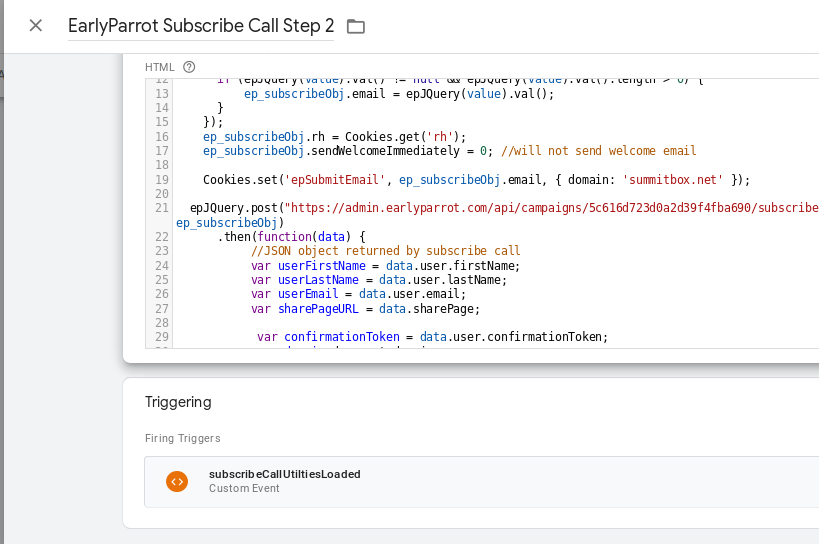
With that set, you are ready to roll.